Understanding Azure Pub/Sub and How to Implement It !
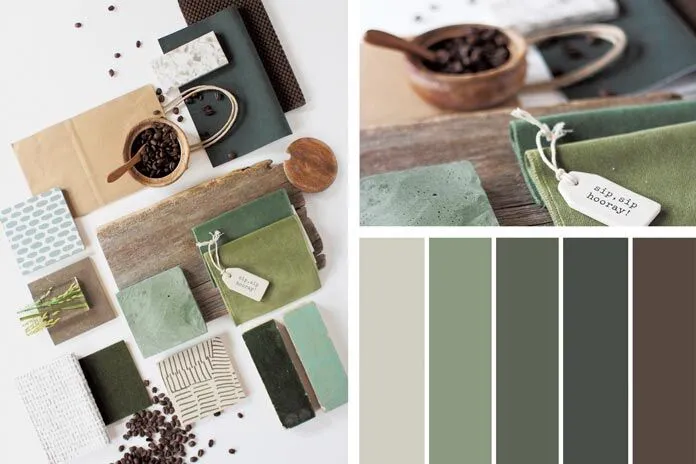
In modern cloud architectures, decoupling components to improve scalability and maintainability is key. One of the most effective messaging patterns for achieving this is Publish-Subscribe (Pub/Sub). Azure provides multiple services to implement Pub/Sub, with Azure Service Bus being one of the most commonly used.
In this post, we’ll explore the Azure Pub/Sub pattern, how it works, and walk through a practical example of how to implement it using Azure Service Bus.
What is the Pub/Sub Pattern?
The Publish-Subscribe (Pub/Sub) pattern is a messaging paradigm that allows publishers (senders of messages) to send messages to a topic, without knowing who or what will consume them. Subscribers, on the other hand, are entities that listen for and process messages from the topic. The key benefit is the decoupling between message producers and consumers—publishers don’t need to know about the subscribers, and vice versa.
The general flow in a Pub/Sub system is as follows:
- Publisher sends a message to a central messaging hub (like a topic).
- Subscribers register to receive messages from the topic, and when a new message is published, it’s delivered to all subscribers.
Azure provides several services that support Pub/Sub, including Azure Service Bus, Azure Event Grid, and Azure Event Hubs. For the purpose of this post, we will focus on Azure Service Bus, a fully managed messaging service that supports both queues and topics, and thus makes it ideal for Pub/Sub architectures.
Why Use Azure Pub/Sub?
Azure Pub/Sub can solve several architectural challenges, such as:
- Scalability: By decoupling the components, systems can be more easily scaled independently.
- Reliability: Service Bus offers durable message storage, ensuring no messages are lost.
- Flexibility: With topics and subscriptions, you can broadcast messages to multiple consumers or filter messages by topics.
- Asynchronous Communication: It allows systems to communicate asynchronously, improving performance and responsiveness.
How Azure Service Bus Implements Pub/Sub
In Azure Service Bus, the topic represents a Pub/Sub messaging channel, and subscriptions are like virtual queues that receive messages sent to that topic. A topic can have multiple subscriptions, and each subscription can filter messages based on various criteria, allowing different consumers to receive different types of messages.
Key Concepts in Azure Service Bus for Pub/Sub:
- Topic: A named resource to which messages are sent by publishers.
- Subscription: A named resource that receives messages sent to a topic. Subscriptions can have filters to receive specific messages.
- Message: A unit of data that is sent from a publisher to a topic and received by subscribers.
- Filter: A set of conditions applied to messages to determine which ones a subscription should receive.
Steps to Implement Azure Pub/Sub Using Service Bus
Let’s walk through how to create a simple Pub/Sub system using Azure Service Bus.
1. Set Up Azure Service Bus Namespace and Topic
First, you need to create a Service Bus Namespace and a Topic within that namespace.
- Go to the Azure Portal.
- In the search bar, type “Service Bus” and click on it.
- Click on + Create to create a new Service Bus namespace.
- Choose a Resource Group, Location, and name your namespace.
- Once the namespace is created, go to it, and under the Entities section, click + Topic to create a new topic.
2. Create Subscriptions
After creating a topic, you can create one or more subscriptions that will receive messages from the topic.
- In the Service Bus Namespace, select the Topic you just created.
- Under the Subscriptions section, click + Subscription to create a new subscription.
- You can create multiple subscriptions if you need different consumers to receive the same message, possibly with filters applied.
3. Publish Messages to the Topic
Next, we will write a simple console application to publish messages to the topic.
Here’s an example in C#:
Replace <Your-Service-Bus-Connection-String>
and <Your-Topic-Name>
with your actual Service Bus connection string and topic name.
using Microsoft.Azure.ServiceBus;
using System;
using System.Text;
using System.Threading.Tasks;
class Program
{
static string ServiceBusConnectionString = "<Your-Service-Bus-Connection-String>";
static string TopicName = "<Your-Topic-Name>";
static ITopicClient topicClient;
static async Task Main(string[] args)
{
topicClient = new TopicClient(ServiceBusConnectionString, TopicName);
// Create a message
string messageBody = "Hello, this is a message for the subscribers!";
Message message = new Message(Encoding.UTF8.GetBytes(messageBody));
// Send the message to the topic
await topicClient.SendAsync(message);
Console.WriteLine($"Message sent: {messageBody}");
await topicClient.CloseAsync();
}
}
4. Create Subscribers to Receive the Messages
Now, let’s create a subscriber that will listen for messages from the topic. You can write a simple C# console application for that as well:
using Microsoft.Azure.ServiceBus;
using System;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
class Program
{
static string ServiceBusConnectionString = "<Your-Service-Bus-Connection-String>";
static string TopicName = "<Your-Topic-Name>";
static string SubscriptionName = "<Your-Subscription-Name>";
static ISubscriptionClient subscriptionClient;
static async Task Main(string[] args)
{
subscriptionClient = new SubscriptionClient(ServiceBusConnectionString, TopicName, SubscriptionName);
// Register message handler
subscriptionClient.RegisterMessageHandler(
async (message, cancellationToken) =>
{
string messageBody = Encoding.UTF8.GetString(message.Body);
Console.WriteLine($"Received message: {messageBody}");
// Complete the message so it is removed from the subscription
await subscriptionClient.CompleteAsync(message.SystemProperties.LockToken);
},
new MessageHandlerOptions(ExceptionReceivedHandler) { MaxConcurrentCalls = 1, AutoComplete = false });
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
await subscriptionClient.CloseAsync();
}
static Task ExceptionReceivedHandler(ExceptionReceivedEventArgs arg)
{
Console.WriteLine($"Exception: {arg.Exception.Message}");
return Task.CompletedTask;
}
}
Replace the placeholders (<Your-Service-Bus-Connection-String>
, <Your-Topic-Name>
, and <Your-Subscription-Name>
) with your actual Service Bus connection details.
5. Filter Messages (Optional)
If you want certain subscribers to receive specific messages, you can add filters to the subscriptions. Filters can be based on message properties, such as the MessageType
or Priority
, to enable fine-grained control over which messages get delivered to which subscribers.
// Add filter when creating subscription in Azure Portal or via Azure CLI/SDK
For example, you might create a filter to only deliver messages of a specific priority to a particular subscriber.
Conclusion
Azure Service Bus is a powerful, fully-managed service that supports the Pub/Sub pattern, allowing you to decouple components in your system, scale them independently, and communicate asynchronously. With just a few steps, you can implement Pub/Sub with Azure Service Bus by setting up topics and subscriptions, and using SDKs to publish and subscribe to messages.
By implementing Azure Pub/Sub, your applications can be more flexible, scalable, and maintainable, while also ensuring reliable message delivery across multiple components.
Next Steps:
- Explore using Azure Event Grid for serverless event-driven architectures.
- Look into Azure Event Hubs for high-throughput event processing scenarios.
- Consider implementing retry policies, dead-letter queues, and other advanced features in Service Bus for production use.