What is microsoft azure function with smple code using c#
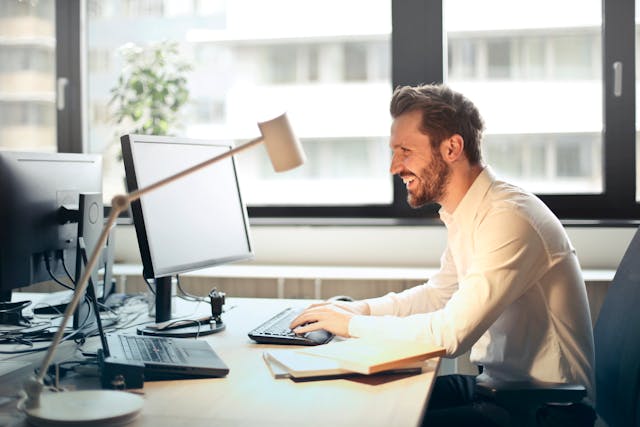
Microsoft Azure Functions is a serverless compute service that allows you to run event-driven code without having to explicitly provision or manage infrastructure. It enables developers to focus on writing the logic for their applications while Azure automatically handles the scaling and infrastructure management. Azure Functions can be triggered by a variety of events, such as HTTP requests, timer events, database changes, and messages from other Azure services.
Key Benefits of Azure Functions
- Serverless Computing: Eliminate the need to manage servers. Azure automatically scales your applications based on demand.
- Event-Driven Execution: Functions can be triggered by various events, making it easy to respond to changes in data, user actions, and system state.
- Cost-Efficiency: Pay only for the compute time your code consumes. This is particularly cost-effective for applications with variable workloads.
- Language Support: Azure Functions supports multiple programming languages, including C#, JavaScript, Python, and more.
- Integration: Easily integrate with other Azure services and third-party APIs to build comprehensive cloud-based solutions.
Getting Started with Azure Functions Using C#
In this section, we’ll walk through the process of creating a simple Azure Function using C#. We’ll build an HTTP-triggered function that responds with a greeting message.
Prerequisites
Before you start, ensure you have the following:
- An Azure account.
- Visual Studio 2019 or later (with the Azure development workload installed).
- Azure Functions Core Tools.
- The Azure CLI.
Step-by-Step Guide
- Create an Azure Function App Project: Open Visual Studio and create a new project. Select the “Azure Functions” template under the “Cloud” category. Name your project and click “Create”.
- Configure Your Function: In the “New Azure Functions Application” dialog, select “HTTP trigger” for the template, and choose “Storage Account (AzureWebJobsStorage)”. Set the authorization level to “Function”.
- Write the Function Code: Visual Studio generates a default HTTP-triggered function. Modify the
Function1.cs
file to customize the function:
using System.IO;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json;
namespace FunctionApp
{
public static class Function1
{
[FunctionName("Function1")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
string name = req.Query["name"];
string requestBody = await new StreamReader(req.Body).ReadToEndAsync();
dynamic data = JsonConvert.DeserializeObject(requestBody);
name = name ?? data?.name;
return name != null
? (ActionResult)new OkObjectResult($"Hello, {name}")
: new BadRequestObjectResult("Please pass a name on the query string or in the request body");
}
}
}
- This function reads a “name” parameter from the query string or request body and returns a greeting message.
- Test Locally: To test the function locally, press
F5
in Visual Studio. This will start the Azure Functions runtime and open a terminal with the local function URL. You can use tools like Postman or a web browser to send a GET request to the URL, appending?name=YourName
to see the response. - Deploy to Azure: Once you’re satisfied with the local testing, right-click the project in Visual Studio and select “Publish”. Follow the prompts to create a new Function App in Azure or select an existing one. After deployment, you can test the function using the URL provided by Azure.
Conclusion
Azure Functions simplifies the process of running small pieces of code in the cloud. Its serverless architecture allows developers to focus on writing code without worrying about infrastructure management. With its event-driven model, it seamlessly integrates with various Azure services and external systems, making it an ideal choice for building scalable, cost-effective applications.
Here’s a recap of the steps to create a simple HTTP-triggered Azure Function using C#:
- Create a new Azure Function App project in Visual Studio.
- Configure the function template.
- Write the function code to process HTTP requests.
- Test the function locally.
- Deploy the function to Azure.
By mastering Azure Functions, you can build responsive, scalable cloud applications efficiently, leveraging the full power of Microsoft’s cloud platform.