Azure Service Bus Short Notes
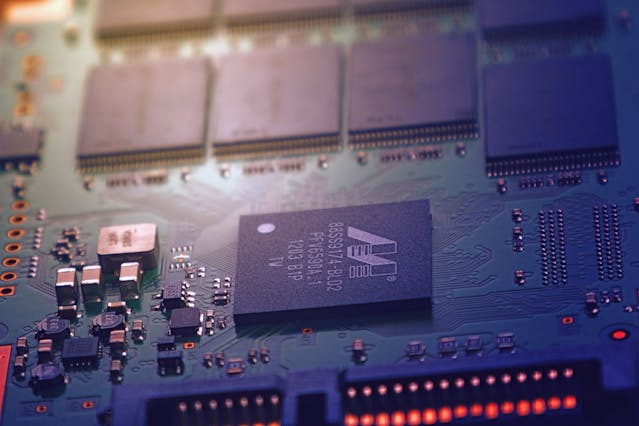
Azure Service Bus is a messaging infrastructure provided by Microsoft Azure that enables the building of scalable and reliable communication between different components of distributed applications. It supports a variety of messaging patterns, such as queues, publish-subscribe, and hybrid messaging, making it a versatile choice for building complex cloud-based solutions. Here’s a concise overview of its key features and components:
Key Features
- Reliable Messaging:
- Ensures message delivery with built-in features for message queuing, delivery guarantees, and retries.
- Scalability:
- Supports the scaling of applications by handling high volumes of messages. Azure Service Bus automatically manages message load and distribution.
- Multiple Messaging Patterns:
- Queues: For point-to-point communication, where messages are sent to a queue and processed by a single receiver.
- Topics and Subscriptions: For publish-subscribe communication, allowing a single message to be delivered to multiple subscribers.
- Advanced Features:
- Sessions: Enables message grouping and stateful processing, facilitating complex workflows and operations.
- Dead-Lettering: Captures messages that cannot be delivered or processed, allowing for later inspection and troubleshooting.
- Duplicate Detection: Prevents the processing of duplicate messages based on a configurable time window.
- Integration with Other Azure Services:
- Seamlessly integrates with Azure Functions, Logic Apps, Event Grid, and other Azure services, enhancing the capabilities of cloud applications.
Components
- Namespaces:
- A logical container for all messaging entities. Each namespace provides a scoping boundary for queues, topics, and subscriptions.
- Queues:
- Standard Queues: For simple message queuing with at-least-once delivery.
- Premium Queues: For higher throughput, lower latency, and more advanced features.
- Topics and Subscriptions:
- Topics: Publish messages to topics, enabling multiple subscriptions to receive copies of the message.
- Subscriptions: Receive messages from a topic. Each subscription can have its own filter criteria.
- Message:
- The fundamental unit of communication. Messages can contain up to 256 KB of data, and they can include properties, labels, and metadata.
Use Cases
- Decoupling Components:
- Use queues and topics to decouple application components, enabling independent development and scaling.
- Load Leveling:
- Buffer messages in queues to manage varying loads and ensure smooth processing without overloading backend services.
- Event-Driven Architectures:
- Implement event-driven architectures using topics and subscriptions to handle real-time events and notifications.
- Hybrid Messaging:
- Integrate on-premises systems with cloud applications using hybrid connections, bridging communication between different environments.
Key Benefits
- Durability and Reliability:
- Ensures message durability with data replication and geo-redundancy, providing high availability and fault tolerance.
- Security:
- Supports authentication and authorization through Azure Active Directory (AAD) and Shared Access Signatures (SAS).
- Monitoring and Diagnostics:
- Provides built-in monitoring, logging, and diagnostic tools, enabling the tracking of message flow and troubleshooting issues.
Example Code Snippet (C#)
Here’s a simple example of sending and receiving messages using Azure Service Bus with C#:
Sending a Message:
using Azure.Messaging.ServiceBus;
using System;
using System.Threading.Tasks;
class Program
{
private const string connectionString = "<your_connection_string>";
private const string queueName = "<your_queue_name>";
static async Task Main(string[] args)
{
await SendMessageAsync();
}
static async Task SendMessageAsync()
{
await using var client = new ServiceBusClient(connectionString);
ServiceBusSender sender = client.CreateSender(queueName);
ServiceBusMessage message = new ServiceBusMessage("Hello, Azure Service Bus!");
await sender.SendMessageAsync(message);
Console.WriteLine("Message sent!");
}
}
Receiving a Message:
using Azure.Messaging.ServiceBus;
using System;
using System.Threading.Tasks;
class Program
{
private const string connectionString = "<your_connection_string>";
private const string queueName = "<your_queue_name>";
static async Task Main(string[] args)
{
await ReceiveMessageAsync();
}
static async Task ReceiveMessageAsync()
{
await using var client = new ServiceBusClient(connectionString);
ServiceBusReceiver receiver = client.CreateReceiver(queueName);
ServiceBusReceivedMessage message = await receiver.ReceiveMessageAsync();
if (message != null)
{
Console.WriteLine($"Received message: {message.Body}");
await receiver.CompleteMessageAsync(message);
}
}
}
Conclusion
Azure Service Bus is a powerful and flexible messaging service that supports a wide range of messaging scenarios, from simple message queuing to complex event-driven architectures. Its reliability, scalability, and integration capabilities make it an essential component for building modern, distributed applications in the cloud. Whether you are developing microservices, integrating systems, or building real-time applications, Azure Service Bus provides the robust messaging infrastructure you need.